If you have data for P-1, will you always have data for all quarters? If not - do you want to start counting at the first non-null quarter or do you want to count for all quarters?
Are you only ever calculating the values for P-1 (Prior), P (Previous) and C (Current)? In other words - only those 12 quarters will be calculated when this code runs?
Here is another way to accomplish this:
Select c.FY
, c.[Year Flag]
, [Quarter NR] = Case When c.[Value] Is Null Then ''
Else cast(row_number() over(Partition By iif(c.[Value] Is Null, 0, 1) Order By c.[FY]) As varchar(2))
End
, c.[Value]
From @Calendar c;
If you only ever calculate for those 3 years...
This version will start counting from the first non-null quarter...for example:
Declare @Calendar Table (
[FY] nvarchar(20) Null
, [Year Flag] nvarchar(10) Null
, [Quarter NR] int Null
, [Value] float Null);
Insert Into @Calendar ([FY], [Year Flag], [Quarter NR], [Value])
Values ('FY19/Q1', 'P-1', '', Null) -- '62')
, ('FY19/Q2', 'P-1', '', Null) --'67')
, ('FY19/Q3', 'P-1', '', 58) --'58')
, ('FY19/Q4', 'P-1', '', 61) --'61')
--Values ('FY19/Q1', 'P-1', '', '62')
-- , ('FY19/Q2', 'P-1', '', '67')
-- , ('FY19/Q3', 'P-1', '', '58')
-- , ('FY19/Q4', 'P-1', '', '61')
, ('FY20/Q1', 'P', '', '23')
, ('FY20/Q2', 'P', '', '41')
, ('FY20/Q3', 'P', '', '80')
, ('FY20/Q4', 'P', '', '89')
, ('FY21/Q1', 'C', '', '64')
, ('FY21/Q2', 'C', '', '86')
, ('FY21/Q3', 'C', '', '11')
, ('FY21/Q4', 'C', '', '15');
Select c.FY
, c.[Year Flag]
, [Quarter NR] = Case When c.[Value] Is Null Then ''
Else cast(row_number() over(Partition By iif(c.[Value] Is Null, 0, 1) Order By c.[FY]) As varchar(2))
End
, c.[Value]
From @Calendar c;
Results:
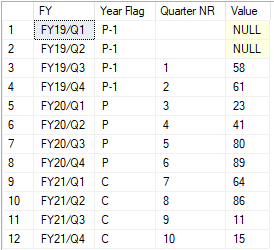