Hi
Please find ur solution
;
WITH abc_cte
AS (
SELECT CreatedAt
,count(orderType) AS countOrders
,sum(PriceSum) AS sumPriceSum
FROM #SampleData
WHERE OrderType <> 2
AND CreatedAt BETWEEN '2015/01/01'
AND '2015/08/20'
GROUP BY CreatedAt
)
SELECT CreatedAt
,countOrders
,sum(sumPriceSum) OVER () AS RoundedPriceSum
FROM abc_cte
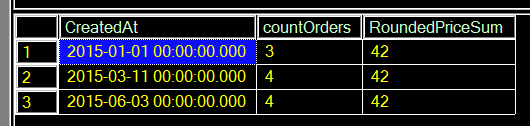
Please find sample data below
/*
IF OBJECT_ID('tempdb.dbo.#SampleData', 'U') IS NOT NULL
drop table #SampleData;
GO
create table #SampleData
(
CreatedAt datetime null,
orderType int null,
PriceSum int null
)
GO
insert into #SampleData select '2015-01-01',4,10
insert into #SampleData select '2015-01-01',3,6
insert into #SampleData select '2015-01-01',1,2
insert into #SampleData select '2015-03-11',5,1
insert into #SampleData select '2015-03-11',3,3
insert into #SampleData select '2015-03-11',5,2
insert into #SampleData select '2015-03-11',3,6
insert into #SampleData select '2015-06-03',4,1
insert into #SampleData select '2015-06-03',4,3
insert into #SampleData select '2015-06-03',1,2
insert into #SampleData select '2015-06-03',3,6
GO
select * from #SampleData
GO
*/
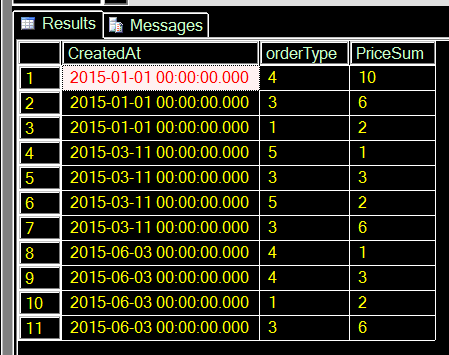